Have you ever automated your trading process using computer programs instead manually opening your demat account and placing buy or sell orders assets in stock market, If you are not, In this article We are going to be learn how to place orders in Angel One to buy a stock with Angel One Smart API using JavaScript code.
Writing computer programs to execute orders in Stock Market(or Crypto Market) when predefined set of conditions are met, known as an Algorithmic Trading(Algo Trading in short). Bringing a profitable trading stragety into code and running that code for to execute orders in market, can bring high possibility to get good profits from our investment.
As a first step to automate our trading process, We are going to learn how do place orders in stock market programmatically.
In this article, We are going to be use Angel One Smart API to programmatically place orders in Angel One with your demat account.
What is Angel One Smart API ?
Angel One providing all the necessary API's to build a complete stock market investment and trading platform from execting orders to manage portfolio's and stream market data through WebSockets. Thanks to Angel One for providing all of this API's for free of cost.
Setting Angel One Smart API Account
To interact with Angel One Smart API, We need to create a account in Angel One Smart API. To create a account, visit to angel one smart api website here.
After creating an account, sign in to your account. Your account dashboard will be look like this,
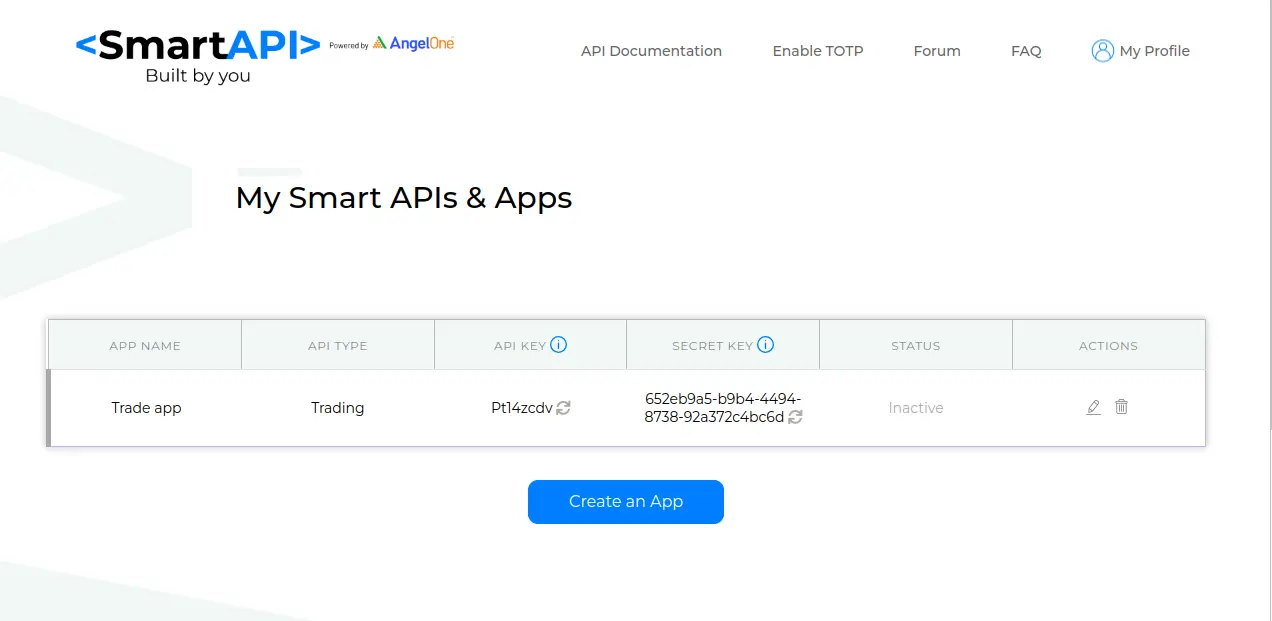
I have created one Trading App in my account. So, In my dashboad there is one app visible. In your case, your dashboard will be empty in first time.
Now Let's create the App by clicking the "Create an App" button in the dashboard page.
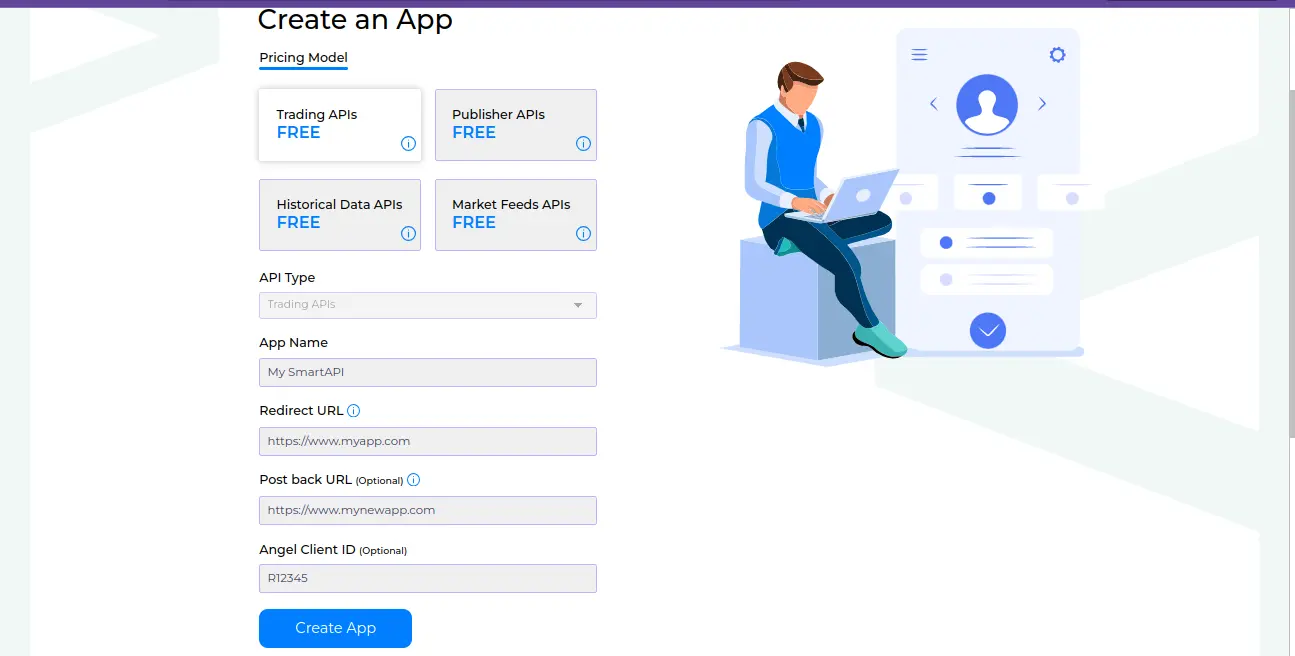
- API Type - Select Trading API, because We are going to place orders
in real time.
- App Name - Give any app name you want, I am giving My Smart API as my app name.
- Redirect URL - According to Angel One Smart API docs, “Redirect URL will receive Access Tokens when you make a Login request to the SmartAPIs”
In our case we are entering “http://127.0.0.1” as our Redirect URL.
- Postback URL - According to Angel One Smart API docs, “This is the URL where you can receive order status for the orders you have placed via APIs”
This is an optional field, So I am leaving it optional.
- Angel Client ID - It is an optional field, if you have a client ID, you can enter this or leave it optional. But, You need to have a Angel One Demat
account to execute orders through Angel One Smart API.
Note: Redirect and Postback URL are useful when you are using any Algo trading platform or If you are creating one trading platform with Angel One Smart API.
After providing these details, Click Create App. It will create an Trading app for us to execute orders.
Now we have trading app created to place orders. With this app's API Key, Secret Key we can execute our orders. But, How can we tell our app to use my demat account to place orders. Angel one gives TOTP Authorization for this purpose.
Enabling TOTP(Time-Base One-Time Password) Authorization
Enable TOTP in angel one smart API dashboard By providing your Angel client Id and PIN. After submitting this details, you will get Secret Key. Keep this Secret Key secretly, So it will used used Authorize your angel one demat account through code.
Now, We have all the things to interact with Angel One to execute trade orders. So, Let's start to write code in JavaSript.
Initialize NodeJS project
Initialize nodejs project with this command, this command will initialize the nodejs project for you.
npm init --yes
And install the packages below.
npm i dotenv smartapi-javascript totp-generator
Create a .env file your project directory, and fill your Angel One credentials here
ANGEL_ONE_USERNAME=
ANGEL_ONE_PIN=
ANGEL_ONE_API_KEY=
ANGEL_ONE_SECRET_KEY=
ANGEL_ONE_TOTP_SECRET=
Placing a Limit Order
In your code, initialize angel one smart api with API Key like below, also use totp-generator to generate totp with your totp secret AngelOne key.
We need to establish a session between your account with smart api before going to call any method that AngelOne provides. So, we establishing a session with generateSession method, and we are making placeOrder request with necessary fields here.
// index.js
require("dotenv").config();
const totp = require("totp-generator");
let { SmartAPI } = require("smartapi-javascript");
let smart_api = new SmartAPI({
api_key: process.env.ANGEL_ONE_API_KEY,
});
const token = totp(process.env.ANGEL_ONE_TOTP_SECRET);
smart_api
.generateSession(
process.env.ANGEL_ONE_USERNAME,
process.env.ANGEL_ONE_PIN,
token
)
.then((data) => {
// place limit order to buy itc stock
return smart_api.placeOrder({
variety: "AMO",
tradingsymbol: "ITC-EQ",
symboltoken: "1660",
transactiontype: "BUY",
exchange: "NSE",
ordertype: "LIMIT",
producttype: "DELIVERY",
duration: "DAY",
price: "459",
quantity: "1",
});
})
.then((data) => {
console.log("Response : ", data);
})
.catch((err) => {
console.log("Error ", err);
});
If you run the above code, it will place a limit order to buy a itc stock for you. Here, I am passing varity field as AMO(After Market Order) order, because I am making this order in after market hour. You need to pass it as "Normal" if you are not placing order in market hours
Each stock in angelone has its own token symbol, you can get find all of the stock’s symbol token here link.
Conclusion